Introduction to MemGraph and BunJS - Building and Querying a Graph Database
In this article, we will explore how to use MemGraph, a powerful open-source graph database, in conjunction with BunJS, a fast and lightweight JavaScript runtime, to build and query a graph database. We will focus on the TokenQuoter project, which utilizes MemGraph to store and query token and pool data.
Prerequisites
- Basic knowledge of graph databases and Cypher query language
- Familiarity with JavaScript and BunJS
- Memgraph and BunJS installed on your system
Step 1: Connecting to the Database
To connect to the Memgraph database using BunJS, you can use the Neo4j driver. First, add and import the neo4j
package and create a driver instance:
import neo4j from 'neo4j-driver';
const uri = 'neo4j://localhost:7687';
const driver = neo4j.driver(uri);
Then, create a session and test the connection:
const session = driver.session();
await session.run('RETURN 1');
await session.close();
Finally, close the driver when you're done:
await driver.close();
Step 2: Understanding the Graph Structure
The TokenQuoter project uses a directed weighted graph to represent tokens, protocols, and chains. The graph consists of nodes (vertices) and relationships (edges). Each node has a unique identifier and properties such as id
, symbol
, address
, and decimals
. Relationships connect nodes and have properties like id
, pool_id
, protocol
, liquidity
, and weight
.
graph TD
A[Token] -->|TRADES_WITH| A
A -->|ON_CHAIN| C[Chain]
A -->|USED_IN| D[Pool]
E[Protocol] -->|CONTAINS| D
A simplified Mermaid diagram for the graph structure
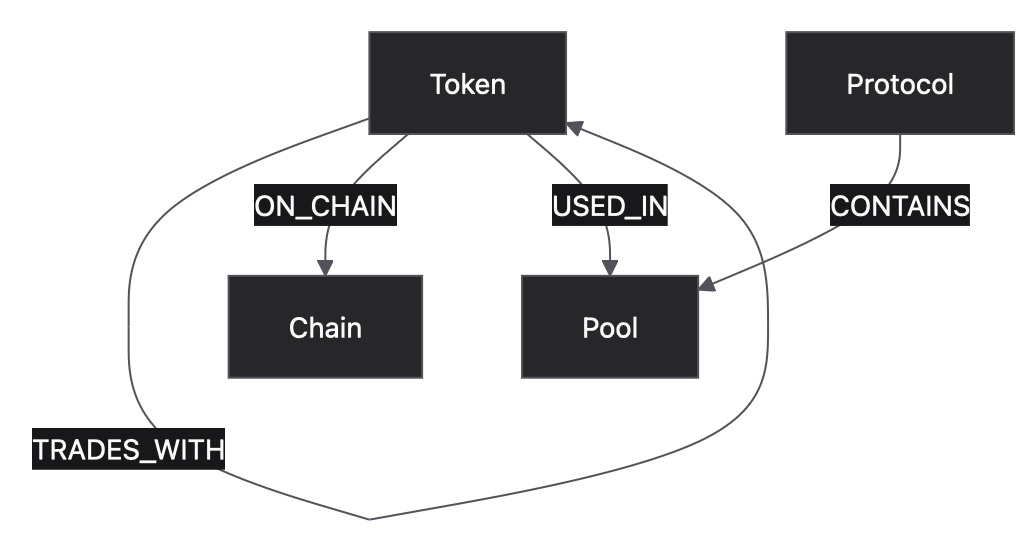
Step 3: Creating Indices
To optimize query performance, create indices on relevant properties:
CREATE INDEX ON :Token(id);
CREATE INDEX ON :Token(symbol);
CREATE INDEX ON :Token(address);
CREATE INDEX ON :Token(chain);
CREATE INDEX ON :Protocol(id);
CREATE INDEX ON :Pool(id);
CREATE INDEX ON :Pool(protocol_system);
CREATE INDEX ON :Chain(id);
CREATE INDEX ON :TRADES_WITH(weight);
CREATE INDEX ON :TRADES_WITH(liquidity);
CREATE INDEX ON :TRADES_WITH(pool_id);
Step 4: Querying the Database
Use Cypher queries to retrieve data from the graph. For example, to get all tokens:
MATCH (t:Token)
RETURN t.symbol, t.address, t.chain
LIMIT 10;
To find direct trading routes between two tokens:
MATCH (t1:Token {symbol: 'WETH'})-[r:TRADES_WITH]->(t2:Token {symbol: 'USDC'})
RETURN t1.symbol, t2.symbol, r.protocol, r.liquidity, r.fee
ORDER BY r.weight ASC
LIMIT 5;
Step 5: Integrating with BunJS App
The TokenQuoter BunJS app integrates with the graph database using the MemgraphClient
class. This class provides methods to connect to and query the database, retrieve tokens and pools, find trading routes, and update order book data.
// Example usage
const graphManager = new GraphManager();
await graphManager.loadFromDB(memgraphClient);
// Find paths between tokens
const paths = await graphManager.findWeightedPaths(
'0xC02aaA39b223FE8D0A0e5C4F27eAD9083C756Cc2', // WETH
'0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48', // USDC
3 // Max hops
);
Step 6: Building a Graph
To build a graph, you need to create nodes and relationships. For example, to create a token node:
CREATE (t:Token {id: 't:ethereum:0xC02aaA39b223FE8D0A0e5C4F27eAD9083C756Cc2', symbol: 'WETH', address: '0xC02aaA39b223FE8D0A0e5C4F27eAD9083C756Cc2', decimals: 18})
To create a relationship between two tokens:
MATCH (t1:Token {symbol: 'WETH'}), (t2:Token {symbol: 'USDC'})
CREATE (t1)-[:TRADES_WITH {pool_id: '0x88e6a0c2ddd26feeb64f039a2c41296fcb3f5640', protocol: 'uniswap_v3', liquidity: 123456789, weight: 1.0 / 123456789}]->(t2)
Step 7: Extracting Value from the Graph
To extract value from the graph, you can use Cypher queries to retrieve specific data. For example, to find the best trading path between two tokens:
MATCH (t1:Token {symbol: 'WETH'})-[routes:TRADES_WITH *wShortest(r, n | r.weight) total_weight]->(t2:Token {symbol: 'USDT'})
WHERE length(routes) <= 3
RETURN t1.symbol, [r IN routes | r.protocol] AS protocols, total_weight, t2.symbol
LIMIT 5;
Conclusion
In this article, we have explored how to use Memgraph and BunJS to build and query a graph database. We have covered the basics of connecting to the database, understanding the graph structure, creating indices, querying the database, integrating with the BunJS app, building a graph, and extracting value from the graph. With this knowledge, you can start building your own graph database applications using Memgraph and BunJS.
Further Reading
- MemGraph documentation: https://memgraph.com/docs/
- BunJS documentation: https://bun.sh/docs